mirror of
https://github.com/MarginaliaSearch/MarginaliaSearch.git
synced 2025-02-24 21:29:00 +00:00
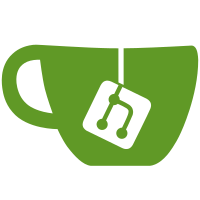
Look, this will make the git history look funny, but trimming unnecessary depth from the source tree is a very necessary sanity-preserving measure when dealing with a super-modularized codebase like this one. While it makes the project configuration a bit less conventional, it will save you several clicks every time you jump between modules. Which you'll do a lot, because it's *modul*ar. The src/main/java convention makes a lot of sense for a non-modular project though. This ain't that.
71 lines
1.6 KiB
Java
71 lines
1.6 KiB
Java
package nu.marginalia.util;
|
|
|
|
import it.unimi.dsi.fastutil.objects.Object2LongOpenHashMap;
|
|
|
|
import java.util.Arrays;
|
|
import java.util.HashMap;
|
|
import java.util.Objects;
|
|
|
|
public class StringPool {
|
|
|
|
private final HashMap<String, String> words;
|
|
private final Object2LongOpenHashMap<String> ages;
|
|
private final int maxCap;
|
|
|
|
long idx;
|
|
|
|
private StringPool(int capacity, int maxCap) {
|
|
this.ages = new Object2LongOpenHashMap<>(capacity);
|
|
this.words = new HashMap<>(capacity);
|
|
this.maxCap = maxCap;
|
|
}
|
|
|
|
public static StringPool create(int capacity) {
|
|
return new StringPool(capacity, capacity * 10);
|
|
}
|
|
|
|
public String internalize(String str) {
|
|
prune();
|
|
|
|
final String ret = words.putIfAbsent(str, str);
|
|
ages.put(ret, idx++);
|
|
|
|
return Objects.requireNonNullElse(ret, str);
|
|
}
|
|
|
|
public String[] internalize(String[] str) {
|
|
|
|
for (int i = 0; i < str.length; i++) {
|
|
str[i] = internalize(str[i]);
|
|
}
|
|
|
|
return str;
|
|
}
|
|
|
|
public void prune() {
|
|
|
|
if (words.size() < maxCap)
|
|
return;
|
|
|
|
long[] ageValues = ages.values().toLongArray();
|
|
Arrays.sort(ageValues);
|
|
|
|
long cutoff = ageValues[ageValues.length - maxCap / 10];
|
|
|
|
words.clear();
|
|
ages.forEach((word, cnt) -> {
|
|
if (cnt >= cutoff) {
|
|
words.put(word, word);
|
|
}
|
|
});
|
|
ages.clear();
|
|
words.forEach((w,w2) -> {
|
|
ages.put(w, idx);
|
|
});
|
|
}
|
|
|
|
public void flush() {
|
|
words.clear();
|
|
}
|
|
}
|