mirror of
https://github.com/MarginaliaSearch/MarginaliaSearch.git
synced 2025-02-24 13:19:02 +00:00
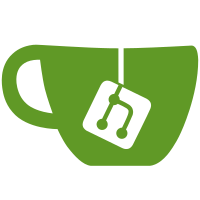
Look, this will make the git history look funny, but trimming unnecessary depth from the source tree is a very necessary sanity-preserving measure when dealing with a super-modularized codebase like this one. While it makes the project configuration a bit less conventional, it will save you several clicks every time you jump between modules. Which you'll do a lot, because it's *modul*ar. The src/main/java convention makes a lot of sense for a non-modular project though. This ain't that.
89 lines
2.4 KiB
Java
89 lines
2.4 KiB
Java
package nu.marginalia.dating;
|
|
|
|
import nu.marginalia.browse.DbBrowseDomainsRandom;
|
|
import nu.marginalia.browse.DbBrowseDomainsSimilarCosine;
|
|
import nu.marginalia.browse.model.BrowseResult;
|
|
import nu.marginalia.db.DomainBlacklist;
|
|
|
|
import java.util.LinkedList;
|
|
|
|
public class DatingSessionObject {
|
|
public final LinkedList<BrowseResult> queue = new LinkedList<>();
|
|
public final LinkedList<BrowseResult> recentlyViewed = new LinkedList<>();
|
|
private BrowseResult current;
|
|
|
|
private static final int MAX_HISTORY_SIZE = 100;
|
|
private static final int MAX_QUEUE_SIZE = 100;
|
|
|
|
public BrowseResult setCurrent(BrowseResult result) {
|
|
current = result;
|
|
return current;
|
|
}
|
|
|
|
public BrowseResult next(DbBrowseDomainsRandom random, DomainBlacklist blacklist) {
|
|
if (queue.isEmpty()) {
|
|
random.getRandomDomains(25, blacklist, 0).forEach(queue::addLast);
|
|
}
|
|
return queue.pollFirst();
|
|
}
|
|
|
|
public BrowseResult nextSimilar(int domainId, DbBrowseDomainsSimilarCosine adjacent, DomainBlacklist blacklist) {
|
|
adjacent.getDomainNeighborsAdjacentCosineRequireScreenshot(domainId, blacklist, 25).forEach(queue::addFirst);
|
|
|
|
while (queue.size() > MAX_QUEUE_SIZE) {
|
|
queue.removeLast();
|
|
}
|
|
|
|
return queue.pollFirst();
|
|
}
|
|
|
|
public void browseForward(BrowseResult res) {
|
|
if (current != null) {
|
|
addToHistory(current);
|
|
}
|
|
setCurrent(res);
|
|
}
|
|
|
|
public void browseBackward(BrowseResult res) {
|
|
if (current != null) {
|
|
addToQueue(current);
|
|
}
|
|
setCurrent(res);
|
|
}
|
|
|
|
public BrowseResult addToHistory(BrowseResult res) {
|
|
recentlyViewed.addFirst(res);
|
|
while (recentlyViewed.size() > MAX_HISTORY_SIZE) {
|
|
recentlyViewed.removeLast();
|
|
}
|
|
return res;
|
|
}
|
|
|
|
public BrowseResult addToQueue(BrowseResult res) {
|
|
queue.addFirst(res);
|
|
while (queue.size() > MAX_QUEUE_SIZE) {
|
|
queue.removeLast();
|
|
}
|
|
return res;
|
|
}
|
|
|
|
public BrowseResult takeFromHistory() {
|
|
return recentlyViewed.pollFirst();
|
|
}
|
|
|
|
public boolean hasHistory() {
|
|
return !recentlyViewed.isEmpty();
|
|
}
|
|
|
|
public boolean isRecent(BrowseResult res) {
|
|
return recentlyViewed.contains(res) || res.equals(current);
|
|
}
|
|
public void resetQueue() {
|
|
queue.clear();
|
|
}
|
|
|
|
public BrowseResult getCurrent() {
|
|
return current;
|
|
}
|
|
}
|