mirror of
https://github.com/MarginaliaSearch/MarginaliaSearch.git
synced 2025-02-24 13:19:02 +00:00
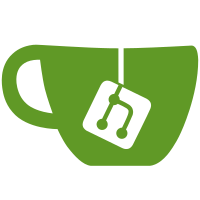
Look, this will make the git history look funny, but trimming unnecessary depth from the source tree is a very necessary sanity-preserving measure when dealing with a super-modularized codebase like this one. While it makes the project configuration a bit less conventional, it will save you several clicks every time you jump between modules. Which you'll do a lot, because it's *modul*ar. The src/main/java convention makes a lot of sense for a non-modular project though. This ain't that.
27 lines
839 B
Java
27 lines
839 B
Java
package nu.marginalia.bigstring;
|
|
|
|
import java.util.Arrays;
|
|
import java.util.concurrent.ThreadLocalRandom;
|
|
|
|
/** To avoid contention on the compression buffer, while keeping allocation churn low,
|
|
* we use a pool of buffers, randomly selected allocated upon invocation
|
|
* <p>
|
|
* @see CompressionBuffer CompressionBuffer
|
|
* */
|
|
public class CompressionBufferPool {
|
|
private static final int BUFFER_COUNT = 16;
|
|
private final CompressionBuffer[] destBuffer;
|
|
|
|
public CompressionBufferPool() {
|
|
destBuffer = new CompressionBuffer[BUFFER_COUNT];
|
|
Arrays.setAll(destBuffer, i -> new CompressionBuffer());
|
|
}
|
|
|
|
/** Get the buffer for the current thread */
|
|
public CompressionBuffer bufferForThread() {
|
|
int idx = ThreadLocalRandom.current().nextInt(0, BUFFER_COUNT);
|
|
|
|
return destBuffer[idx];
|
|
}
|
|
}
|