mirror of
https://github.com/MarginaliaSearch/MarginaliaSearch.git
synced 2025-02-25 05:38:59 +00:00
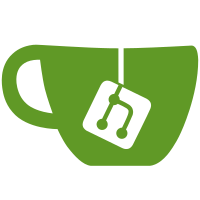
Look, this will make the git history look funny, but trimming unnecessary depth from the source tree is a very necessary sanity-preserving measure when dealing with a super-modularized codebase like this one. While it makes the project configuration a bit less conventional, it will save you several clicks every time you jump between modules. Which you'll do a lot, because it's *modul*ar. The src/main/java convention makes a lot of sense for a non-modular project though. This ain't that.
45 lines
1.8 KiB
Java
45 lines
1.8 KiB
Java
package nu.marginalia.model;
|
|
|
|
import nu.marginalia.model.EdgeUrl;
|
|
import org.junit.jupiter.api.Test;
|
|
|
|
import java.net.URISyntaxException;
|
|
|
|
import static org.junit.jupiter.api.Assertions.assertEquals;
|
|
|
|
class EdgeUrlTest {
|
|
|
|
@Test
|
|
public void testHashCode() throws URISyntaxException {
|
|
System.out.println(new EdgeUrl("https://memex.marginalia.nu").hashCode());
|
|
}
|
|
|
|
@Test
|
|
public void testFragment() throws URISyntaxException {
|
|
assertEquals(
|
|
new EdgeUrl("https://memex.marginalia.nu/"),
|
|
new EdgeUrl("https://memex.marginalia.nu/#here")
|
|
);
|
|
}
|
|
@Test
|
|
public void testParam() throws URISyntaxException {
|
|
System.out.println(new EdgeUrl("https://memex.marginalia.nu/index.php?id=1").toString());
|
|
System.out.println(new EdgeUrl("https://memex.marginalia.nu/showthread.php?id=1&count=5&tracking=123").toString());
|
|
}
|
|
@Test
|
|
void urlencodeFixer() throws URISyntaxException {
|
|
System.out.println(EdgeUrl.urlencodeFixer("https://www.example.com/#heredoc"));
|
|
System.out.println(EdgeUrl.urlencodeFixer("https://www.example.com/%-sign"));
|
|
System.out.println(EdgeUrl.urlencodeFixer("https://www.example.com/%22-sign"));
|
|
System.out.println(EdgeUrl.urlencodeFixer("https://www.example.com/\n \"huh\""));
|
|
}
|
|
|
|
@Test
|
|
void testParms() throws URISyntaxException {
|
|
System.out.println(new EdgeUrl("https://search.marginalia.nu/?id=123"));
|
|
System.out.println(new EdgeUrl("https://search.marginalia.nu/?t=123"));
|
|
System.out.println(new EdgeUrl("https://search.marginalia.nu/?v=123"));
|
|
System.out.println(new EdgeUrl("https://search.marginalia.nu/?m=123"));
|
|
System.out.println(new EdgeUrl("https://search.marginalia.nu/?follow=123"));
|
|
}
|
|
} |