mirror of
https://github.com/MarginaliaSearch/MarginaliaSearch.git
synced 2025-02-24 13:19:02 +00:00
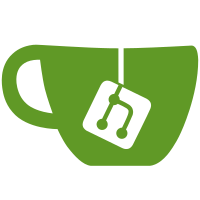
Look, this will make the git history look funny, but trimming unnecessary depth from the source tree is a very necessary sanity-preserving measure when dealing with a super-modularized codebase like this one. While it makes the project configuration a bit less conventional, it will save you several clicks every time you jump between modules. Which you'll do a lot, because it's *modul*ar. The src/main/java convention makes a lot of sense for a non-modular project though. This ain't that.
58 lines
1.7 KiB
Java
58 lines
1.7 KiB
Java
package nu.marginalia.service;
|
|
|
|
import com.google.inject.Inject;
|
|
import com.zaxxer.hikari.HikariDataSource;
|
|
import lombok.SneakyThrows;
|
|
import org.slf4j.Logger;
|
|
import org.slf4j.LoggerFactory;
|
|
|
|
import java.sql.SQLException;
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
import java.util.concurrent.TimeUnit;
|
|
|
|
public class NodeConfigurationWatcher {
|
|
private static final Logger logger = LoggerFactory.getLogger(NodeConfigurationWatcher.class);
|
|
private final HikariDataSource dataSource;
|
|
|
|
private volatile List<Integer> queryNodes = new ArrayList<>();
|
|
|
|
@Inject
|
|
public NodeConfigurationWatcher(HikariDataSource dataSource) {
|
|
this.dataSource = dataSource;
|
|
|
|
var watcherThread = new Thread(this::pollConfiguration, "Node Configuration Watcher");
|
|
watcherThread.setDaemon(true);
|
|
watcherThread.start();
|
|
}
|
|
|
|
@SneakyThrows
|
|
private void pollConfiguration() {
|
|
for (;;) {
|
|
List<Integer> goodNodes = new ArrayList<>();
|
|
|
|
try (var conn = dataSource.getConnection()) {
|
|
var stmt = conn.prepareStatement("""
|
|
SELECT ID FROM NODE_CONFIGURATION
|
|
WHERE ACCEPT_QUERIES AND NOT DISABLED
|
|
""");
|
|
var rs = stmt.executeQuery();
|
|
while (rs.next()) {
|
|
goodNodes.add(rs.getInt(1));
|
|
}
|
|
}
|
|
catch (SQLException ex) {
|
|
logger.error("Error polling node configuration", ex);
|
|
}
|
|
|
|
queryNodes = goodNodes;
|
|
|
|
TimeUnit.SECONDS.sleep(10);
|
|
}
|
|
}
|
|
|
|
public List<Integer> getQueryNodes() {
|
|
return queryNodes;
|
|
}
|
|
}
|