mirror of
https://github.com/RoboSats/robosats.git
synced 2024-12-13 19:06:26 +00:00
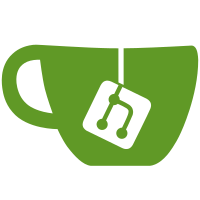
Added the Order model with a rough approxiation to the fields needed. The field status can be read lineally as the progression trough the app pipeline. The view serves POSTs requests to enter new orders into the db.
40 lines
1.3 KiB
Python
40 lines
1.3 KiB
Python
from rest_framework import generics, status
|
|
from rest_framework.views import APIView
|
|
from rest_framework.response import Response
|
|
|
|
from .serializers import OrderSerializer, MakeOrderSerializer
|
|
from .models import Order
|
|
|
|
# Create your views here.
|
|
|
|
class MakeOrder(APIView):
|
|
serializer_class = MakeOrderSerializer
|
|
|
|
def post(self,request, format=None):
|
|
|
|
serializer = self.serializer_class(data=request.data)
|
|
print(serializer)
|
|
if serializer.is_valid():
|
|
otype = serializer.data.get('type')
|
|
currency = serializer.data.get('currency')
|
|
amount = serializer.data.get('amount')
|
|
premium = serializer.data.get('premium')
|
|
satoshis = serializer.data.get('satoshis')
|
|
|
|
#################
|
|
# TODO
|
|
# query if the user is already a maker or taker, return error
|
|
|
|
# Creates a new order in db
|
|
order = Order(
|
|
type=otype,
|
|
currency=currency,
|
|
amount=amount,
|
|
premium=premium,
|
|
satoshis=satoshis)
|
|
order.save()
|
|
|
|
if not serializer.is_valid():
|
|
return Response(status=status.HTTP_400_BAD_REQUEST)
|
|
|
|
return Response(OrderSerializer(order).data, status=status.HTTP_201_CREATED) |