mirror of
https://github.com/RoboSats/robosats.git
synced 2025-01-11 08:41:34 +00:00
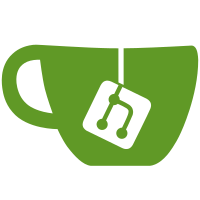
* Re-init new tradebox * Wip progress on OrderDetails * Wip 2 OrderDetails * Fix multiple requests on load * Add functional Order page * Fixes order page * Fix delete storage * Fix order page style * Add Public order prompt * Add paused order prompt * Add expired prompt * Create statusToContract logics * Move fetch order loop to Main * Add payout and wait prompts * Fix order fetch on badOrder * Fix styles * Add chat and dispute prompts * Add remaining prompts * Fix style * Add notifications component * Fix take order style, add more notifications * Add page title notification * Add more notifications and small tradebox fixes * Small fixes * Small fixes to routing failure prompt * Remove old trade box * Add bad take order
77 lines
2.0 KiB
TypeScript
77 lines
2.0 KiB
TypeScript
import React from 'react';
|
|
import { useTranslation } from 'react-i18next';
|
|
import { Box, Tooltip } from '@mui/material';
|
|
import { Order } from '../../models';
|
|
import { LoadingButton } from '@mui/lab';
|
|
|
|
interface CancelButtonProps {
|
|
order: Order;
|
|
onClickCancel: () => void;
|
|
openCancelDialog: () => void;
|
|
openCollabCancelDialog: () => void;
|
|
loading: boolean;
|
|
}
|
|
|
|
const CancelButton = ({
|
|
order,
|
|
onClickCancel,
|
|
openCancelDialog,
|
|
openCollabCancelDialog,
|
|
loading = false,
|
|
}: CancelButtonProps): JSX.Element => {
|
|
const { t } = useTranslation();
|
|
|
|
const showCancelButton =
|
|
(order.is_maker && [0, 1, 2].includes(order.status)) || [3, 6, 7].includes(order.status);
|
|
const showCollabCancelButton = [8, 9].includes(order.status) && !order.asked_for_cancel;
|
|
const noConfirmation =
|
|
(order.is_maker && [0, 1, 2].includes(order.status)) || (order.is_taker && order.status === 3);
|
|
|
|
return (
|
|
<Box>
|
|
{showCancelButton ? (
|
|
<Tooltip
|
|
placement='top'
|
|
enterTouchDelay={500}
|
|
enterDelay={700}
|
|
enterNextDelay={2000}
|
|
title={
|
|
noConfirmation
|
|
? t('Cancel order and unlock bond instantly')
|
|
: t('Unilateral cancelation (bond at risk!)')
|
|
}
|
|
>
|
|
<div>
|
|
<LoadingButton
|
|
size='small'
|
|
loading={loading}
|
|
variant='outlined'
|
|
color='secondary'
|
|
onClick={noConfirmation ? onClickCancel : openCancelDialog}
|
|
>
|
|
{t('Cancel')}
|
|
</LoadingButton>
|
|
</div>
|
|
</Tooltip>
|
|
) : (
|
|
<></>
|
|
)}
|
|
{showCollabCancelButton ? (
|
|
<LoadingButton
|
|
size='small'
|
|
loading={loading}
|
|
variant='outlined'
|
|
color='secondary'
|
|
onClick={openCollabCancelDialog}
|
|
>
|
|
{t('Collaborative Cancel')}
|
|
</LoadingButton>
|
|
) : (
|
|
<></>
|
|
)}
|
|
</Box>
|
|
);
|
|
};
|
|
|
|
export default CancelButton;
|