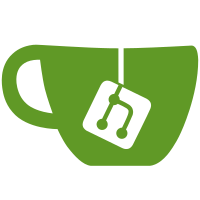
- Fixed featured image upload and handling - Added detailed debug logging - Cleaned up fallback image functionality - Updated README.md with new MIT license - Added .gitignore file - Fixed image reposting functionality - Improved error handling and logging - Added proper WordPress coding standards
146 lines
5.2 KiB
JavaScript
146 lines
5.2 KiB
JavaScript
jQuery(document).ready(function($) {
|
|
// Post status container element
|
|
const $statusContainer = $('.bluesky-post-status');
|
|
|
|
// Helper function to display status messages
|
|
function updateStatus(message, type = 'info') {
|
|
const $status = $(`<div class="notice notice-${type} is-dismissible"><p>${message}</p></div>`);
|
|
$statusContainer.prepend($status);
|
|
|
|
// Add dismiss button functionality
|
|
$status.find('.notice-dismiss').on('click', function() {
|
|
$status.fadeOut(300, function() { $(this).remove(); });
|
|
});
|
|
|
|
// Auto dismiss after 5 seconds for success messages
|
|
if (type === 'success') {
|
|
setTimeout(() => {
|
|
$status.fadeOut(300, function() { $(this).remove(); });
|
|
}, 5000);
|
|
}
|
|
}
|
|
|
|
// Handle immediate posting
|
|
$('.bluesky-share-post').on('click', function(e) {
|
|
e.preventDefault();
|
|
const $button = $(this);
|
|
const postId = $button.data('post-id');
|
|
const nonce = $button.data('nonce');
|
|
|
|
// Disable button and show loading state
|
|
$button.prop('disabled', true)
|
|
.addClass('updating-message')
|
|
.text(blueskyAdmin.strings.publishing);
|
|
|
|
$.ajax({
|
|
url: blueskyAdmin.ajaxUrl,
|
|
type: 'POST',
|
|
data: {
|
|
action: 'bluesky_post_now',
|
|
post_id: postId,
|
|
nonce: nonce
|
|
},
|
|
success: function(response) {
|
|
if (response.success) {
|
|
updateStatus(response.data.message, 'success');
|
|
location.reload();
|
|
} else {
|
|
updateStatus(response.data.message, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.retry);
|
|
}
|
|
},
|
|
error: function(xhr, status, error) {
|
|
updateStatus(blueskyAdmin.strings.error, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.retry);
|
|
}
|
|
});
|
|
});
|
|
|
|
// Handle retry posting
|
|
$('.bluesky-retry-post').on('click', function(e) {
|
|
e.preventDefault();
|
|
const $button = $(this);
|
|
const postId = $button.data('post-id');
|
|
const nonce = $button.data('nonce');
|
|
|
|
// Disable button and show loading state
|
|
$button.prop('disabled', true)
|
|
.addClass('updating-message')
|
|
.text(blueskyAdmin.strings.retrying);
|
|
|
|
$.ajax({
|
|
url: blueskyAdmin.ajaxUrl,
|
|
type: 'POST',
|
|
data: {
|
|
action: 'bluesky_post_now',
|
|
post_id: postId,
|
|
nonce: nonce
|
|
},
|
|
success: function(response) {
|
|
if (response.success) {
|
|
updateStatus(response.data.message, 'success');
|
|
location.reload();
|
|
} else {
|
|
updateStatus(response.data.message, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.retry);
|
|
}
|
|
},
|
|
error: function(xhr, status, error) {
|
|
updateStatus(blueskyAdmin.strings.error, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.retry);
|
|
}
|
|
});
|
|
});
|
|
|
|
// Handle reposting
|
|
$('.bluesky-repost').on('click', function(e) {
|
|
e.preventDefault();
|
|
const $button = $(this);
|
|
const postId = $button.data('post-id');
|
|
const nonce = $button.data('nonce');
|
|
|
|
if (!confirm(blueskyAdmin.strings.confirmRepost)) {
|
|
return;
|
|
}
|
|
|
|
$button.prop('disabled', true)
|
|
.addClass('updating-message')
|
|
.text(blueskyAdmin.strings.reposting);
|
|
|
|
$.ajax({
|
|
url: blueskyAdmin.ajaxUrl,
|
|
type: 'POST',
|
|
data: {
|
|
action: 'bluesky_post_now',
|
|
post_id: postId,
|
|
nonce: nonce,
|
|
repost: true
|
|
},
|
|
success: function(response) {
|
|
if (response.success) {
|
|
updateStatus(response.data.message, 'success');
|
|
location.reload();
|
|
} else {
|
|
updateStatus(response.data.message, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.repost);
|
|
}
|
|
},
|
|
error: function(xhr, status, error) {
|
|
updateStatus(blueskyAdmin.strings.error, 'error');
|
|
$button.prop('disabled', false)
|
|
.removeClass('updating-message')
|
|
.text(blueskyAdmin.strings.repost);
|
|
}
|
|
});
|
|
});
|
|
}); |